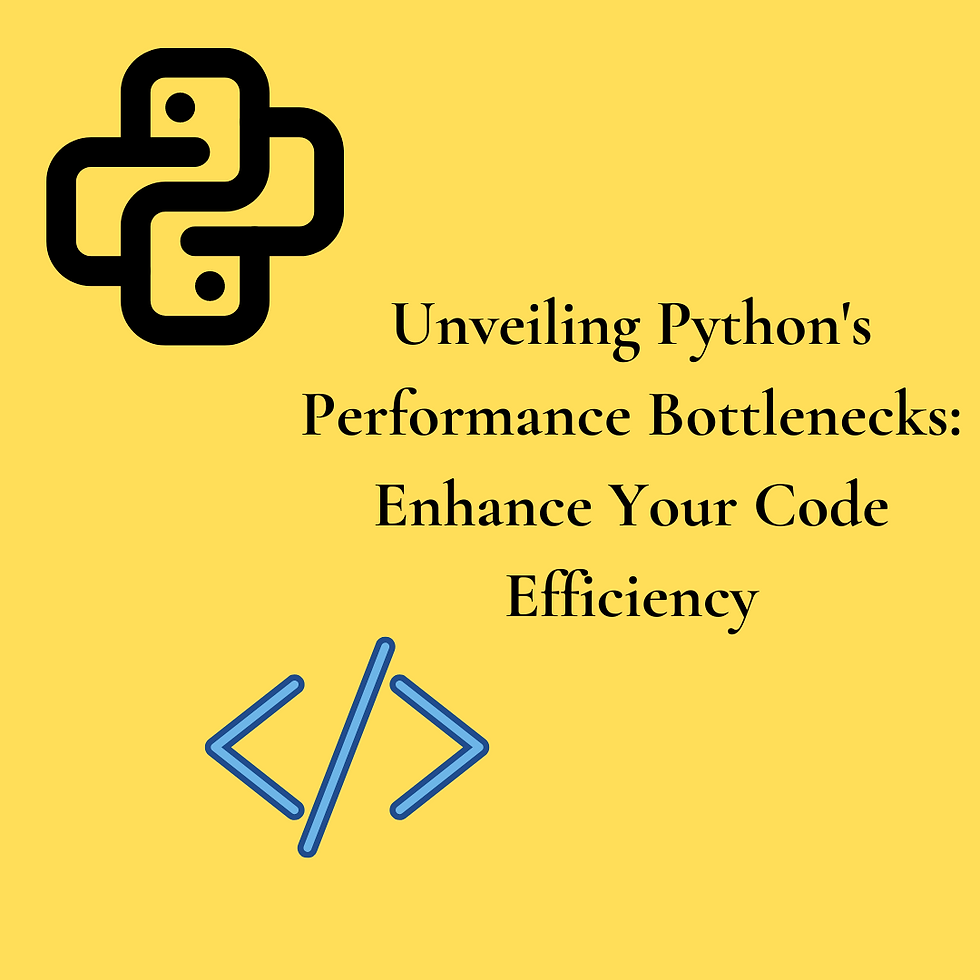
Introduction
Python is renowned for its simplicity and versatility, making it a preferred choice for developers across various domains. However, this flexibility comes at a cost—Python can sometimes be slower compared to languages like C++ or Java. Understanding the common performance bottlenecks in Python is crucial for optimizing code efficiency and improving overall performance. In this article, we'll delve into the key areas where Python code can lag and explore strategies to mitigate these bottlenecks.
1. Looping Over Large Data Structures
The Pitfalls of Loops
Iterating over large data structures like lists or dictionaries in Python can significantly impact performance, especially when using traditional for loops. Each iteration introduces overhead, leading to slower execution times.
Solution: List Comprehensions and Generators
Utilize list comprehensions and generator expressions whenever possible. These constructs offer concise and efficient ways to process data without the performance overhead of traditional loops.
For example:
# Traditional for loop
squares = []
for i in range(10):
squares.append(i**2)
# Using list comprehension
squares = [i**2 for i in range(10)]
Similarly, generators can be employed to lazily evaluate expressions, saving memory and improving performance when dealing with large datasets.
2. Excessive Memory Usage
The RAM Conundrum
Python's dynamic typing and automatic memory management can sometimes lead to excessive memory usage, especially when dealing with large objects or long-running processes. This can result in slower performance and even memory errors for resource-intensive applications.
Solution: Efficient Memory Management
Adopt memory-efficient data structures like NumPy arrays or data streaming libraries such as Dask for handling large datasets. Additionally, consider optimizing memory usage by avoiding unnecessary object creation and releasing resources explicitly when they're no longer needed, using techniques like context managers or the del statement.
3. Inefficient String Manipulation
String Shenanigans
String manipulation operations like concatenation (+ operator) within loops can be inefficient in Python due to the immutable nature of strings. Each concatenation creates a new string object, leading to excessive memory overhead and slower execution.
Leveraging Join Method and String Formatting
Prefer using the join method instead of string concatenation within loops to efficiently build strings. Additionally, utilize string formatting techniques like f-strings or the format method for improved readability and performance. For example:
# Inefficient string concatenation
result = ''
for word in words:
result += word
# Efficient string joining
result = ''.join(words)
4. Suboptimal I/O Operations
IO Woes
Inefficient I/O operations, such as reading from or writing to files, network sockets, or databases, can introduce significant bottlenecks in Python applications, especially when handling large volumes of data.
Asynchronous I/O and Buffered I/O
Harness asynchronous I/O capabilities offered by libraries like asyncio or aiohttp for concurrent I/O operations, enabling better utilization of system resources and improved performance. Additionally, leverage buffered I/O operations to minimize overhead associated with frequent read/write calls, enhancing overall I/O performance.
5. Lack of Parallelism and Concurrency
The Single-Threaded Conundrum
Python's Global Interpreter Lock (GIL) can inhibit true parallelism, limiting the effectiveness of multi-threading for CPU-bound tasks. This can result in suboptimal performance, especially on multi-core systems.
Multiprocessing and Concurrent Futures
Employ the multiprocessing module or concurrent futures for parallel execution of CPU-bound tasks, leveraging multiple processes or threads to fully utilize available CPU cores. Alternatively, explore alternative Python implementations like Jython or IronPython, which offer better support for parallelism without the GIL limitations.
Conclusion
Understanding and addressing performance bottlenecks is essential for maximizing the efficiency of Python code, especially in a Python Training Course in Nagpur, Gwalior, Lucknow, Delhi, Noida, and all cities in India. By optimizing looping constructs, managing memory effectively, improving string manipulation techniques, optimizing I/O operations, and harnessing parallelism and concurrency, developers can significantly enhance the performance of their Python applications. By applying the strategies outlined in this article, you can unlock the full potential of Python and ensure smooth and efficient execution of your code.
Comments