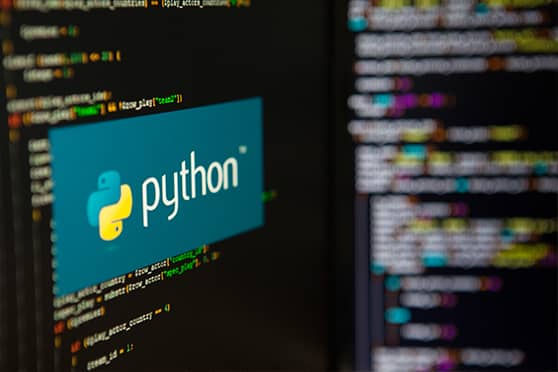
Object-Oriented Programming (OOP) is a programming paradigm that structures software using objects and classes, enhancing modularity and reusability. Python, known for its simplicity and versatility, provides robust support for OOP, making it an excellent choice for both beginners and experienced developers.
Key Concepts of OOP
To effectively use OOP in Python, it's essential to understand its core concepts: classes, objects, inheritance, encapsulation, and polymorphism.
Classes and Objects
A class serves as a blueprint for creating objects. It defines a set of attributes and methods that the created objects will possess. An object is an instance of a class, containing actual data and the methods to manipulate that data.
For example, imagine a Dog class representing dogs. The class might include attributes such as the dog's name and age, and methods like barking or fetching. When you create an object of this class, such as a specific dog named Buddy, you are creating an instance of the Dog class.
Inheritance
Inheritance allows a class to inherit attributes and methods from another class. This promotes code reusability and establishes a hierarchical relationship between classes. The class that inherits is called the subclass or derived class, and the class from which it inherits is known as the superclass or base class.
For instance, if you have a base class called Animal, you could create a subclass Dog that inherits from Animal. This way, Dog would automatically have all the attributes and methods defined in Animal, and you could add more specific features to Dog.
Encapsulation
Encapsulation is the concept of bundling data (attributes) and methods (functions) that operate on the data into a single unit or class. It also involves restricting direct access to some of the object's components, which is a means of preventing unintended interference and misuse of the data.
In practice, encapsulation is achieved by using private and protected access modifiers. This way, you can control how the data within an object is accessed and modified, ensuring that it is done in a controlled manner.
Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon, even though they share the same name. This means a single function or method can work in different ways depending on the context.
For example, a method called speak could be used in both a Dog class and a Cat class, but each implementation would provide different behavior suitable for each type of animal. This allows for flexible and extendable code.
Benefits of OOP in Python
Modularity: By dividing the program into distinct classes and objects, OOP makes it easier to manage and maintain the code.
Reusability: Inheritance and polymorphism enable code reuse, reducing redundancy and improving efficiency.
Scalability: OOP facilitates the development of scalable applications, as new functionalities can be added with minimal changes to existing code.
Productivity: By providing a clear structure for the code, OOP can enhance developer productivity and collaboration.
Real-World Applications
OOP is widely used in various real-world applications, including:
Web Development: Frameworks like Django and Flask utilize OOP principles to create scalable and maintainable web applications.
Game Development: OOP allows developers to create complex game structures with reusable objects and components.
Data Science: Libraries like pandas and scikit-learn use OOP to provide powerful data manipulation and machine learning tools.
GUI Applications: Toolkits like Tkinter enable the creation of graphical user interfaces using OOP concepts.
Best Practices for OOP in Python
Keep Classes Focused: Each class should have a single responsibility or focus. This makes the code more understandable and easier to maintain.
Use Inheritance Wisely: While inheritance is powerful, overusing it can lead to complicated and fragile code. Prefer composition over inheritance where appropriate.
Encapsulate Data: Protect the internal state of objects by using private and protected attributes. Provide public methods to interact with the object's data.
Leverage Polymorphism: Use polymorphism to design flexible and extendable code that can handle new requirements with minimal changes.
Follow Naming Conventions: Adhere to Python's naming conventions for classes, methods, and attributes to improve code readability and consistency.
Conclusion
Object-oriented programming in Python offers a structured approach to software development, promoting code modularity, reusability, and scalability. By understanding and applying the core principles of OOP—classes, objects, inheritance, encapsulation, and polymorphism—developers can create robust and maintainable applications. Whether you're building a web application, a game, or a data analysis tool, OOP provides the framework to write clear, efficient, and scalable Python code. For those interested in mastering these skills, Python courses in Lucknow, Gwalior, Delhi, Noida, and all locations in India offer comprehensive training to enhance your programming expertise.
Kommentare