Mastering State Management with Redux
- k86874248
- Jun 1, 2024
- 4 min read
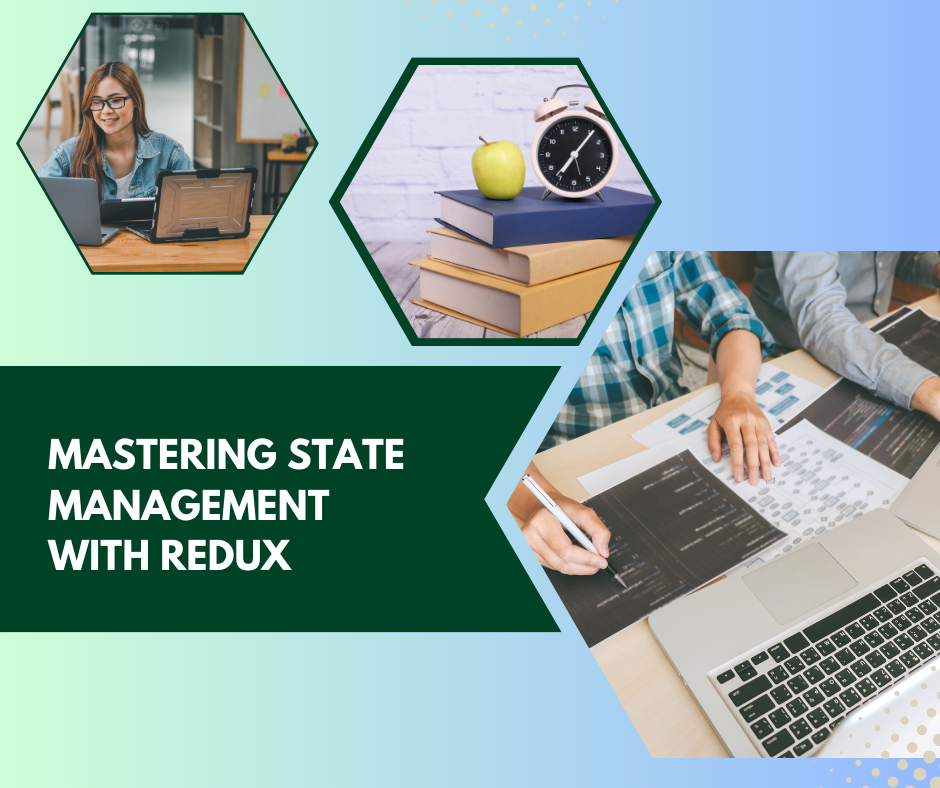
State management is a crucial aspect of building robust and scalable web applications. As applications grow in complexity, managing state efficiently becomes increasingly challenging. Redux, a popular state management library for JavaScript applications, offers a predictable and structured way to handle application state.
Understanding State Management
Before diving into Redux, it's important to understand what state management entails. In web applications, "state" refers to the data that influences the behavior and rendering of the user interface (UI). Managing this state can be tricky, especially as applications grow and the state becomes more complex.
State management libraries like Redux help by providing a systematic approach to handle the state, ensuring that data flows in a predictable manner and is easily traceable. This predictability makes debugging and testing more straightforward.
What is Redux?
Redux is a state management library that follows the principles of Flux architecture. It was created by Dan Abramov and Andrew Clark to manage the state of JavaScript applications. Redux is particularly popular in the React ecosystem, but it can be used with any JavaScript framework or library.
Core Principles of Redux
Redux operates on three core principles:
Single Source of Truth: The entire state of the application is stored in a single JavaScript object called the "store." This centralizes the state, making it easier to manage and debug.
State is Read-Only: The state can only be changed by dispatching actions. Actions are plain JavaScript objects that describe the change. This principle ensures that the state changes in a predictable manner.
Changes are Made with Pure Functions: To specify how the state tree is transformed by actions, you write pure functions called "reducers." A pure function is one that returns the same output for the same input and does not cause side effects.
Key Concepts in Redux
Understanding the key concepts of Redux is essential to mastering state management. Let's explore these concepts in detail:
Store
The store is the central hub that holds the state of the application. It provides methods to access the state, dispatch actions, and register listeners for state changes.
Actions
Actions are plain JavaScript objects that represent an intention to change the state. Every action must have a type property, which is a string that describes the action. Actions can also include additional data that is necessary to perform the state change.
Reducers
Reducers are pure functions that take the current state and an action as arguments and return a new state. They determine how the state should change in response to an action. Because reducers are pure functions, they do not modify the existing state but return a new state object.
Dispatch
Dispatch is the method used to send actions to the store. When an action is dispatched, the store passes it to the reducer, which calculates the new state.
Selectors
Selectors are functions that extract specific pieces of state from the store. They provide an abstraction layer, making it easier to access and compute derived state.
Benefits of Using Redux
Redux offers several benefits that make it a powerful tool for state management:
Predictability
Since state changes are centralized and follow a strict structure, the state of the application becomes predictable. This predictability simplifies debugging and testing.
Maintainability
Redux encourages a clear separation of concerns. By splitting the state management logic into actions, reducers, and selectors, the codebase becomes more organized and maintainable.
Debugging
Redux has excellent debugging tools, such as Redux DevTools, which allow developers to
inspect every state change and action in the application. This visibility helps identify and resolve issues quickly.
Scalability
As applications grow, managing state can become increasingly complex. Redux's structured approach scales well with the application, making it easier to handle large and intricate state trees.
Best Practices for Using Redux
To make the most of Redux, consider following these best practices:
Keep State Normalized
Store the state in a normalized form to avoid redundancy and ensure data consistency. This involves structuring the state in a way that reduces nested data and makes it easier to update.
Use Middleware
Middleware extends Redux with custom functionality, such as handling asynchronous actions or logging. Popular middleware includes Redux Thunk for asynchronous actions and Redux Logger for logging state changes.
Leverage Immutable Data Structures
Immutable data structures prevent direct modifications to the state, ensuring that state changes are predictable and easier to track. Libraries like Immutable.js can be used to enforce immutability.
Write Unit Tests
Unit tests are crucial for ensuring the reliability of the state management logic. Write tests for actions, reducers, and selectors to verify their behavior.
Modularize Reducers
As the application grows, a single reducer can become unwieldy. Split the reducers into smaller, focused functions and combine them using the combineReducers utility provided by Redux.
Avoid Overusing Redux
While Redux is powerful, it should not be used for everything. Local component state and React's Context API can handle state that does not need to be globally accessible or is not complex enough to require Redux.
Conclusion
Mastering state management with Redux requires understanding its core principles and key concepts. By centralizing the state, enforcing immutability, and using pure functions, Redux provides a predictable and scalable way to manage the application state. Following best practices will help you leverage the full potential of Redux, leading to maintainable and robust applications. Whether you're building a small project or a large-scale application, Redux can be a valuable tool in your development toolkit. Enroll in a Full Stack Developer Course in Lucknow, Gwalior, Delhi, Noida, and all locations in India to enhance your skills and learn how to effectively use Redux in your projects.
Comments