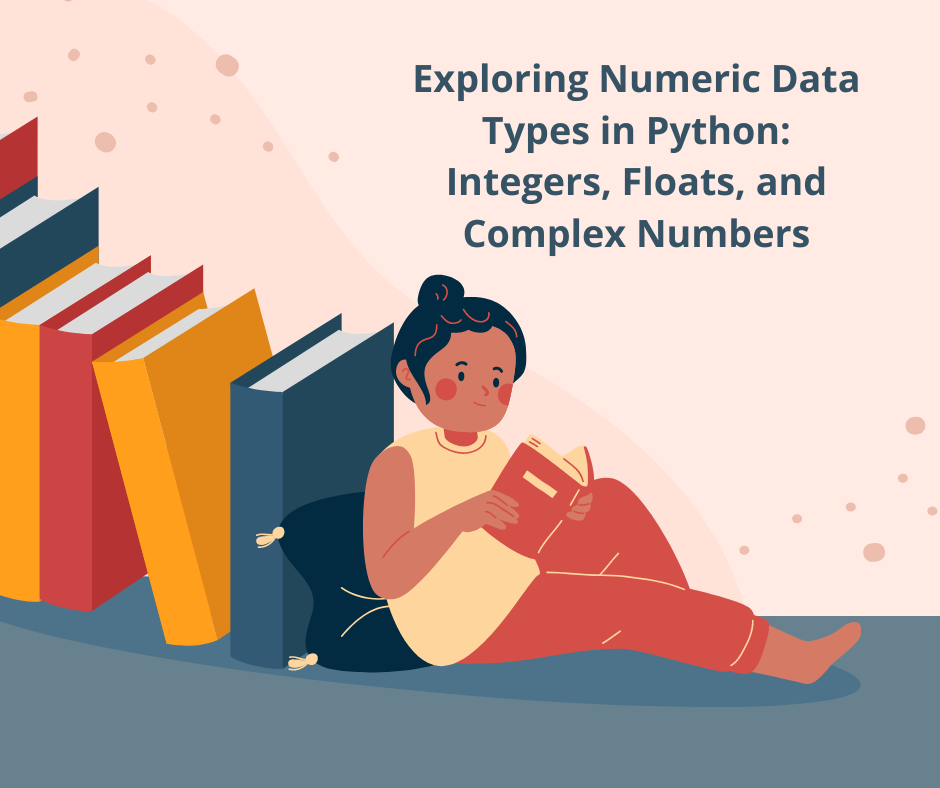
Python is a powerful and versatile programming language known for its simplicity and readability. Among its many features, Python offers a rich set of numeric data types that support a wide range of mathematical and computational tasks. Understanding these numeric data types is fundamental for anyone looking to delve into Python programming. In this article, we will explore three primary numeric data types in Python: integers, floats, and complex numbers.
1. Integers
Definition and Characteristics
Integers in Python are whole numbers, both positive and negative, including zero. They are represented by the int class and can be of arbitrary precision, meaning they can grow as large as the memory allows, unlike in some other programming languages that limit the size of integers.
Usage
Integers are used in a variety of scenarios, such as counting, indexing, and performing arithmetic operations. Here are some examples of integer operations:
# Basic integer operations
a = 10
b = 3
print(a + b) # Addition: 13
print(a - b) # Subtraction: 7
print(a * b) # Multiplication: 30
print(a // b) # Floor Division: 3
print(a % b) # Modulus: 1
print(a ** b) # Exponentiation: 1000
Type Conversion
Python allows easy conversion between integers and other numeric types:
# Converting a float to an integer
float_num = 10.7
int_num = int(float_num) # Results in 10
# Converting a string to an integer
str_num = "123"
int_from_str = int(str_num) # Results in 123
2. Floats
Definition and Characteristics
Floats, or floating-point numbers, represent real numbers and are used for decimal and fractional values. They are represented by the float class and follow the IEEE 754 standard for floating-point arithmetic, which can lead to precision issues in some cases.
Usage
Floats are essential for scientific computations, financial calculations, and any application requiring precision. Here are some basic operations with floats:
# Basic float operations
x = 5.75
y = 2.5
print(x + y) # Addition: 8.25
print(x - y) # Subtraction: 3.25
print(x * y) # Multiplication: 14.375
print(x / y) # Division: 2.3
print(x ** y) # Exponentiation: 45.254833995939045
Precision and Rounding
Due to the way floats are stored in memory, they can sometimes yield unexpected results:
# Example of precision issue
print(0.1 + 0.2) # Results in 0.30000000000000004
# Correcting precision with rounding
result = 0.1 + 0.2
print(round(result, 2)) # Results in 0.3
Type Conversion
Floats can be converted to integers and vice versa:
# Converting an integer to a float
int_num = 10
float_num = float(int_num) # Results in 10.0
# Converting a string to a float
str_float = "123.45"
float_from_str = float(str_float) # Results in 123.45
3. Complex Numbers
Definition and Characteristics
Complex numbers in Python represent numbers with both real and imaginary parts, following the form a + bj, where a is the real part and b is the imaginary part. They are represented by the complex class.
Usage
Complex numbers are particularly useful in advanced scientific and engineering computations, such as signal processing and control systems. Here are some examples:
# Creating complex numbers
c1 = 2 + 3j
c2 = 1 - 4j
print(c1 + c2) # Addition: (3-1j)
print(c1 - c2) # Subtraction: (1+7j)
print(c1 * c2) # Multiplication: (14-5j)
print(c1 / c2) # Division: (-0.7+0.4j)
Accessing Real and Imaginary Parts
You can access the real and imaginary parts of a complex number using the .real and .imag attributes:
# Accessing parts of a complex number
c = 4 + 5j
print(c.real) # Results in 4.0
print(c.imag) # Results in 5.0
Conjugates
The conjugate of a complex number is obtained by changing the sign of the imaginary part:
# Finding the conjugate
c = 3 + 4j
conjugate = c.conjugate() # Results in (3-4j)
Type Conversion
Complex numbers can be created from integers or floats:
# Creating a complex number from an integer and a float
int_num = 7
float_num = 2.5
complex_num = complex(int_num, float_num) # Results in (7+2.5j)
Conclusion
Understanding and utilizing Python's numeric data types—integers, floats, and complex numbers—is crucial for effective programming and problem-solving. Each type has its unique characteristics and applications, making Python a robust language for both basic and advanced computations. By mastering these data types through Python classes in Lucknow, Gwalior, Delhi, Noida, and all cities in India, you can harness the full potential of Python in a wide range of domains, from everyday arithmetic to complex scientific calculations.
Comments