Introduction:
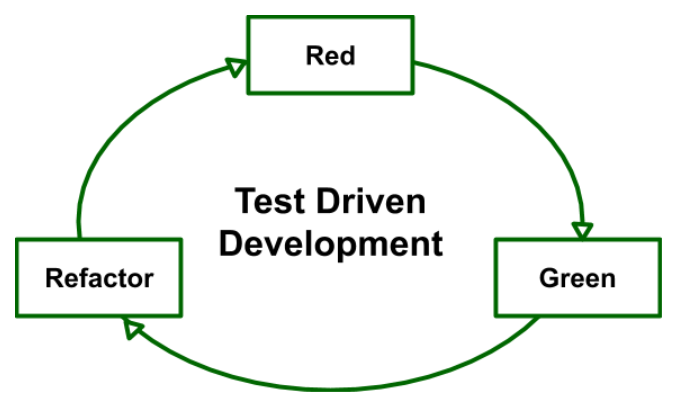
Test-Driven Development (TDD) is an approach to software development where tests are written before the actual code. It emphasizes defining code functionality through tests before implementing it. Java, being one of the most popular programming languages, is widely utilized for TDD. In this guide, we'll delve into the basics of Test-Driven Development in Java, its benefits, and how to get started.
Understanding Test-Driven Development:
What is Test-Driven Development?
Test-Driven Development is a software development process that follows a simple cycle: Write a test, write the code to pass the test, and refactor the code. This cycle is often referred to as "Red-Green-Refactor".
The Three Laws of TDD:
First Law: Write a failing test before writing any production code.
Second Law: Write just enough production code to make the failing test pass.
Third Law: Refactor the code to improve its design while keeping the tests passing.
Getting Started with Test-Driven Development in Java:
Setting Up Your Environment:
Install Java Development Kit (JDK) on your system.
Choose a Java IDE (Integrated Development Environment) such as Eclipse, IntelliJ IDEA, or NetBeans.
Install a testing framework like JUnit or TestNG. In this guide, we'll use JUnit, which is widely adopted in the Java community.
Writing Your First Test:
Let's say we want to implement a simple calculator class. We'll start by writing a test for the additional functionality.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(4, calculator.add(2, 2));
}
}
Running the Test:
When the test is run, it will fail because the add method hasn't been implemented yet.
Writing the Production Code:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Running the Test Again:
After implementing the add method, rerun the test. It should pass now.
Refactoring:
Once the test passes, refactor the code if necessary. However, since our code is already simple, there's no need for further refactoring at this stage.
Benefits of Test-Driven Development:
Improved Code Quality: TDD encourages writing clean, modular, and maintainable code.
Faster Feedback Loop: Tests provide instant feedback on the correctness of your code, helping catch bugs early.
Increased Confidence: A comprehensive suite of tests gives developers confidence when making changes or refactoring code.
Better Design: TDD promotes a design-first approach, leading to more modular and flexible architectures.
Documentation: Tests serve as executable documentation, illustrating how the code should behave.
Challenges of Test-Driven Development:
Initial Learning Curve: TDD requires a shift in mindset and may be challenging for developers new to the concept.
Time Investment: Writing tests upfront may seem time-consuming initially, but it pays off in the long run by reducing debugging time.
Test Maintenance: As the codebase evolves, tests may need updating to reflect changes, requiring additional effort.
Conclusion:
Test-Driven Development in Java is a powerful approach to software development that emphasizes writing tests before implementing functionality. By following the Red-Green-Refactor cycle and leveraging tools like JUnit, developers can improve code quality, catch bugs early, and design more robust systems. While TDD requires initial investment and may present challenges, the benefits it offers in terms of code quality, confidence, and maintainability make it a valuable practice for any Java developer. Start small, practice regularly, and experience the benefits of TDD firsthand in your Java projects. Additionally, consider exploring Java courses in Gwalior, Indore, Lucknow, Delhi, Noida, and all locations in India to deepen your understanding and proficiency in this powerful programming language.
Comments